Programs
Jassembly » Devlog
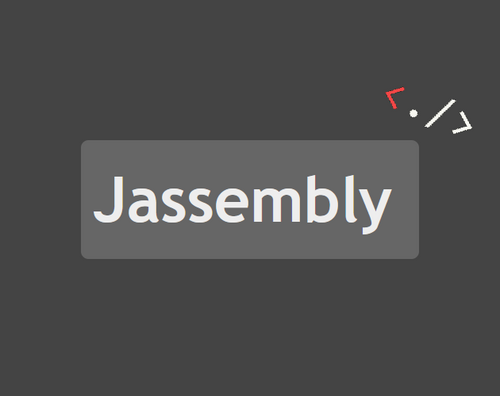
Hello, and welcome fellow devs!
If you want to share your program: This is the right place to do so! :)
Jassembly
This is a programming language, similar to assembly.
Status | In development |
Category | Tool |
Author | Barni - 07 |
Genre | Simulation |
Tags | 2D, coding, computer, javascript, language, program, programming-language, tech, tool |
More posts
- Update V3.01 day ago
- Update V2.08 days ago
Comments
Log in with itch.io to leave a comment.